FX Translator [SCRIPT]
Posted: Mon Oct 14, 2013 7:07 am
Hello fellow community!
A request from pandaFox in the torch thread led me to design a quick script that can translate fxs, lights and particles around.
Video: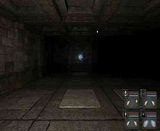
Script:
- Setup instructions in the script header
- It is framerate independent, the effect will always move at the correct speed as long as framerate stays above 10fps.
- Don't worry if you don't understand the code, it has been tweaked for performance, not readability. Just spawn your fx and call the translateFx function the script takes care of the rest.
Enjoy!
A request from pandaFox in the torch thread led me to design a quick script that can translate fxs, lights and particles around.
Video:
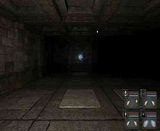
Script:
SpoilerShow
Code: Select all
--[[
Diarmuid's FX Translator script
V 1.11
put this in a script entity named fxTranslator
put a call to fxTranslator.update() from the onDrawGui.
Call fxTranslator.translateFx(fxId, direction, distance, speed)
- distance is expressed in meters (1 tile = 3 meters)
- speed is expressed in meters/sec (3 speed = 1s per tile. Fireball is 7.5, Lightning Bolt is 10)
- direction can be a facing number (0-3) or a {x, y, z} vector table, each value going from 0-1.
]]
fxTable = {};
lastFrameTime = 0;
dt = 0;
function updateDt()
local t = getStatistic("play_time");
dt = t - lastFrameTime;
lastFrameTime = t;
end
function update()
updateDt();
for i = #fxTable, 1, -1 do
local fxData = fxTable[i];
if fxData then
local fxEntity = findEntity(fxData[1]);
if fxEntity then
local translate = dt * 3 / fxData[2];
fxEntity:translate(fxData[3] * translate, fxData[4] * translate, fxData[5] * translate);
fxData[7] = fxData[7] + translate;
if fxData[7] > fxData[6] then
table.remove(fxTable, i);
end
else
table.remove(fxTable, i);
end
end
end
end
function translateFx(fxId, direction, distance, speed)
local dx, dy, dz;
if type(direction) == "table" then
dx, dy, dz = unpack(direction);
else
dx, dz = getForward(direction);
dy = 0;
dz = -dz;
end
local interval = 1/speed*3;
fxTable[#fxTable+1] = {fxId, interval, dx, dy, dz, distance, 0};
end
- It is framerate independent, the effect will always move at the correct speed as long as framerate stays above 10fps.
- Don't worry if you don't understand the code, it has been tweaked for performance, not readability. Just spawn your fx and call the translateFx function the script takes care of the rest.
Enjoy!