(The scripts are updated after i find an error!)
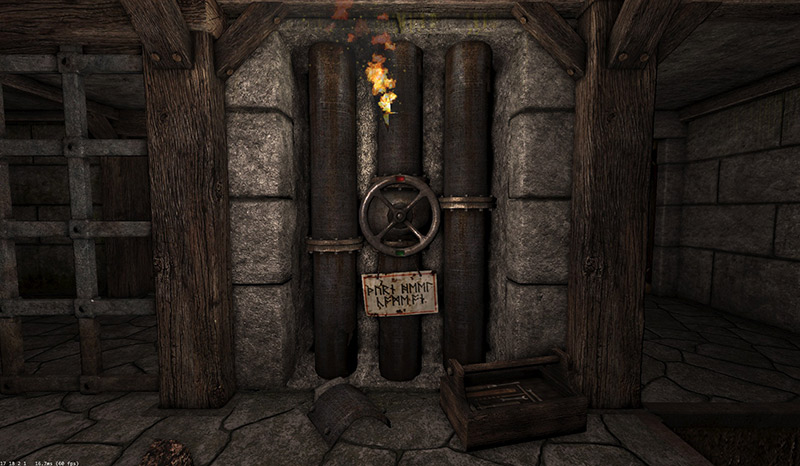
While burning it can´t fixed, so close the valve..
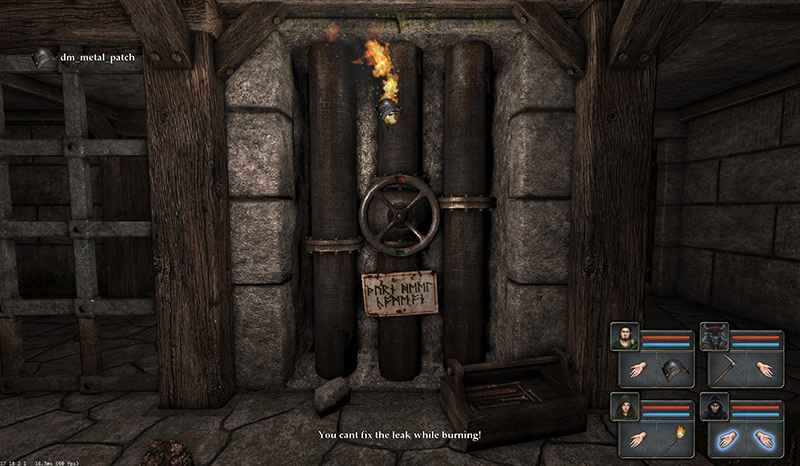
The metal_patch is inserted, then click with toolbox on it - fixed!
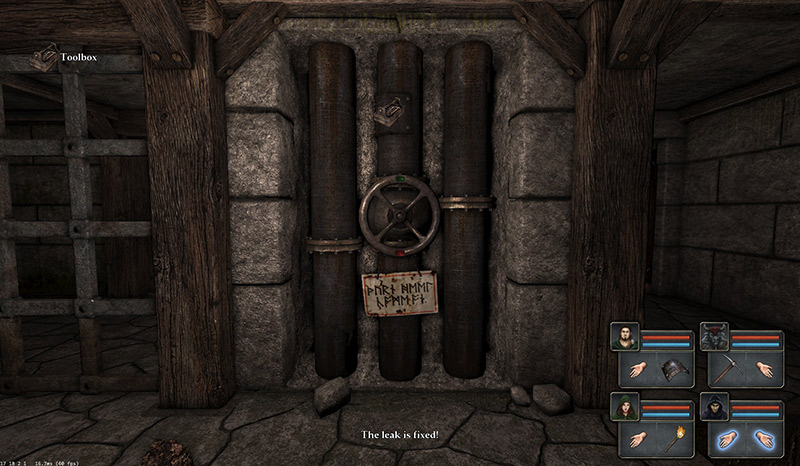
Now the valve work normal as a 'lever' asset!
Youtube:The fixing process in action!
The idea:
- party finds a tube with handwheel valve (Example: operate an invisible engine via gas steam)
- the valve is reqired to trigger next events (Examples: open a door, dry a flooded level entrance..)
- but the tube has a leakage; gas streams out and burns if the wheel valve is open (activated)
- party must fix the leakage with a metal patch and tools (they have to find the items of course^^)
- for fixing, the handwheel valve has to be closed (deactivated) and metal_patch must attached to the tube.
- one click with the toolbox on the tube leakage while metal_patch is inserted will fix the tube.
- if handwheel is again opened (activated), the burning gas steam + sound is gone and event is triggered.
Parts used:
- tube with leakage asset
- handwheel asset def. as lever
- metal_pach_tube asset def. as decoration
- alcove object placed exactly at leakage position
- 1x metal_patch, 1x toolbox items
- custom sounds: 2x for handwheel, 1x steam burning, 1x fixing sound
- 1x lightsource over the leak, 1x invisible lightsource with burning particle system, placed inside the leak
- 4x script - steam playsound, handwheel actions, alcove actions(yet placed in objects.lua), handwheel sound-replacer
- 1x timer for looped non-loop sound-playback (thx Isaac!)
- 1x counter to make 'tubefixed' event from alcove noticeable to the handwheel script
All works well now - a bit noobish scripted i guess^^
Lots of ifs and elseif checks to prevent errors..
Scripts work in editor and in compiled game file!
The Scripts:
This script is for replace lever sounds;
placed in dungeon without connections.
Script Name: leverSndSet
Code: Select all
----begin -----------------------------------------------
-- Set connectors to all levers in dungeon (thx to Isaac!)
for l = 1, getMaxLevels() do
for e in allEntities(l) do
if e.class == "Lever" then
e:addConnector('any', 'leverSndSet', 'playLvSnd')
end
end
end
-- The function to play custom sounds
function playLvSnd(dmDungLev)
-- define and set variables
local lvState = dmDungLev:getLeverState()
local lvLevl = dmDungLev.level
local lvNam = dmDungLev.name
local lvFac = dmDungLev.facing
local lvPosX = dmDungLev.x
local lvPosY = dmDungLev.y
-- play sounds for each state
if lvNam == 'dm_lever_wheel' and lvState == 'activated' then
playSoundAt("leverwheel_open", lvLevl, lvPosX, lvPosY)
elseif lvNam == 'dm_lever_wheel' and lvState == 'deactivated' then
playSoundAt("leverwheel_close", lvLevl, lvPosX, lvPosY)
end
end
Script to place in dungeon for the handwheel valve,
added connector from handwheel lever.
initial state: deactivated
Action on connector: any
Script Name: steamChx
Code: Select all
----begin -----------------------------------------------
function togglActivity(self)
local triggerCif = counter_2:getValue()
local lvState = self:getLeverState()
local lvLevl = self.level
local lvNam = self.name
local lvFac = self.facing
local lvPosX = self.x
local lvPosY = self.y
local fxEmit = "dm_conduit_fx_emitter"
local fxLight = "dm_conduit_fx_light"
local fxEmId = "cond_fx_emit_1"
local fxLiId = "cond_fx_light_1"
local timerNam = timer_1
if lvState == 'activated' and triggerCif == 0 then
timerNam:activate()
spawn(fxEmit, lvLevl, lvPosX, lvPosY, lvFac, fxEmId)
spawn(fxLight, lvLevl, lvPosX, lvPosY, lvFac, fxLiId)
elseif lvState == 'activated' and triggerCif > 0 then
-- begin place further actions here!
if dm_door_steamop:isClosed() then
dm_door_steamop:open()
end
-- end place further actions here!
elseif lvState == 'deactivated' then
for i in entitiesAt(lvLevl,lvPosX,lvPosY) do
if i.id == fxEmId or i.id == fxLiId then
i:destroy()
end
end
if timerNam:isActivated() then
timerNam:deactivate()
end
end
end
----end -----------------------------------------------
Code: Select all
---begin------------------------------------------
defineObject{
name = "dm_conduit_leak_socket",
class = "Alcove",
anchorPos = vec(-0.0826, 2.2, 0.9),
anchorRotation = vec(0, 0, 0),
targetPos = vec(-0.0826, 2.2, 0.175),
targetSize = vec(0.06, 0.1, 0.1),
placement = "wall",
editorIcon = 92,
onInsertItem = function (self, item)
local countNam = counter_2
local triggerCif = countNam:getValue()
if triggerCif > 0 then
return false
end
local metPat = "dm_metal_patch"
local tooBox = "dm_toolbox"
local fxEmit = "dm_conduit_fx_emitter"
local patCon = "dm_patch_conduit"
local patCid = "dm_patch_conduit_1"
local fxEmId = "cond_fx_emit_1"
local fxLiId = "cond_fx_light_1"
for i in entitiesAt(self.level,self.x,self.y) do
if i.id == fxEmId and item.name == tooBox or i.id == fxEmId and item.name == metPat then
hudPrint("You can not fix the leak while burning!")
return false
elseif i.id == fxEmId then
return false
end
end
local px = 0
for i in self:containedItems() do
if i.name == metPat then
px = px + 1
end
end
if item.name ~= metPat or item.name ~= tooBox then
setMouseItem(spawn(item.name))
end
if item.name == metPat and px == 0 then
patchIsIn = true
return item.name
elseif item.name == metPat and px == 1 then
setMouseItem(spawn(metPat))
patchIsIn = true
end
if item.name == tooBox and px == 1 then
toolIsIn = true
setMouseItem(spawn(tooBox))
elseif item.name == tooBox and px == 0 then
setMouseItem(spawn(tooBox))
toolIsIn = false
end
if patchIsIn == true and toolIsIn == true and px == 1 then
playSoundAt("dm_repair",self.level,self.x,self.y)
spawn(patCon,self.level,self.x,self.y,self.facing,patCid)
for z in self:containedItems() do
if z.name == metPat then
z:destroy()
end
end
hudPrint("The leak is fixed!")
countNam:increment()
end
end
}
---end------------------------------------------
Connected to 'timer_1' ; its settings:
timer interval: 1.356, initial state: stopped
Script Name: timedLoopSnd
Code: Select all
----begin -----------------------------------------------
function loopedSound(self)
playSoundAt('dm_steamburn',dm_lever_wheel_1.level, dm_lever_wheel_1.x, dm_lever_wheel_1.y)
end
----end -----------------------------------------------
initial value: 0
No connector
And the sound definitions in 'sounds.lua':
Code: Select all
defineSound{
name = "lever",
filename = "mod_assets/dmcsb_pack/samples/env/lever_nil.wav",
loop = false,
volume = 1,
minDistance = 1,
maxDistance = 8,
}
defineSound{
name = "leverwheel_open",
filename = "mod_assets/dmcsb_pack/samples/env/dm_valvesqueak_open.wav",
loop = false,
volume = 1,
minDistance = 1,
maxDistance = 9,
}
defineSound{
name = "leverwheel_close",
filename = "mod_assets/dmcsb_pack/samples/env/dm_valvesqueak_close.wav",
loop = false,
volume = 1,
minDistance = 1,
maxDistance = 9,
}
defineSound{
name = "dm_steamburn",
filename = "mod_assets/dmcsb_pack/samples/env/dm_steamburn.wav",
loop = false,
volume = 0.5,
minDistance = 1,
maxDistance = 8,
}
defineSound{
name = "dm_repair",
filename = "mod_assets/dmcsb_pack/samples/env/dm_repair.wav",
loop = false,
volume = 1.2,
minDistance = 1,
maxDistance = 6,
}