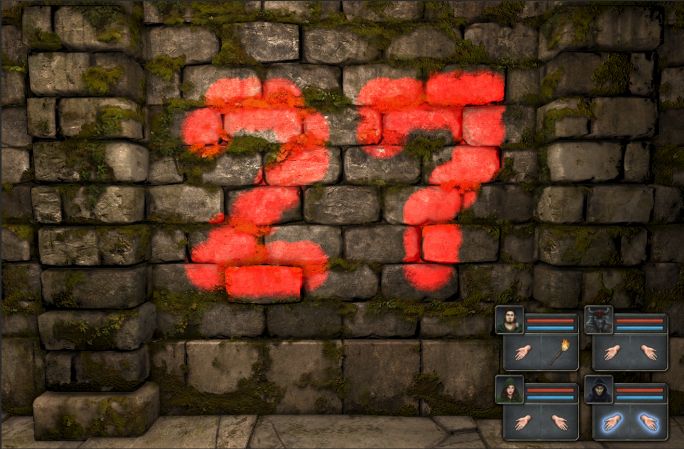
Code: Select all
---------------------------------------------------------------------------------------
--- LED display: version 1.4.3 12-9-2014 Written by Lark ---
--- Much thanks to: Lmaoboat ---
---------------------------------------------------------------------------------------
---
--- Light Parameters
---
displayed = self.level --the initial number displayed by the script
wall = self.facing --adjust wall used for display from script's facing; i.e. opposite = (self.facing + 2) % 4
initallyOn = true --specify if the light is initially on
vertical_spacing = .17 --vertical LED spacing
horizontal_spacing = .15 --horizontal LED spacing; center, left, & right values are based on this number being .15
height = 3 --height above the floor for the top row of LEDs
center = -.3 --start of row offset for single digit displays
left = -.85 --start of row offset for left digit
right = .25 --start of row offset for right digit
--- Usage:
---
--- 1. drop this script into a square facing the wall where the display is desired (or adjust "wall" above)
--- 2. set parameters to suit: displayed, wall, and initiallyOn for basic parameters
--- 3. on() to display the initial value
--- 4. off() to turn off lights
--- 5. add() to increment by one
--- 6. sub() to decrement by one
--- 7. display(number) to set the display to a new number
--- 8. do not call digit() directly
---
--- Version 1.4.3:
--- * Fixed issue of storing objects in a non-local table; now just store the object name (Thanks Isaac)
--- * Updated "off" routine to handle names instead of objects.
--- * Removed bad "null" and replaced with "nil" - mixed up LUA and another language...
--- * Improved error checking in the "off" routine - avoids crashes in strange circumstances
---
--- Create a LED panel for numbers 0 - 99. LED grid is:
---
-- 00 01 02 03 04
-- 05 06 07 08 09
-- 10 11 12 13 14
-- 15 16 17 18 19
-- 20 21 22 23 24
-- 25 26 27 28 29
-- 30 31 32 33 34
digits = {
{1,2,3,5,9,10,14,15,19,20,24,25,29,31,32,33},
{6,2,7,12,17,22,27,31,32,33},
{5,1,2,3,9,14,18,22,26,30,31,32,33,34},
{5,1,2,3,9,14,18,17,16,24,29,33,32,31,25},
{0,5,10,15,16,17,18,19,3,8,13,23,28,33},
{4,3,2,1,0,5,10,15,16,17,18,24,29,33,32,31,30},
{9,3,2,1,5,10,15,20,25,31,32,33,29,24,18,17,16},
{5,0,1,2,3,4,9,14,18,22,27,32},
{10,5,1,2,3,9,14,18,17,16,20,25,31,32,33,29,24},
{25,31,32,33,29,24,19,14,9,3,2,1,5,10,16,17,18}
}
led = {nil}
cos = math.cos(1.5707963267949 * wall)
sin = math.sin(1.5707963267949 * wall)
tz = 1.3
---
--- divide into digits and display in correct sector
---
function display(number)
if number == nil then return end
local b = number % 10
local a = (number - b) / 10
off()
if a > 0 then
digit(a, left)
digit(b, right)
else
digit(b, center)
end
displayed = number
end
---
--- display a digit of the number at the given alignment and light set
---
function digit(number, align)
local dix = number + 1
for ix = 1, 35 do
local pix = digits[dix][ix]
if pix == nil then return end
local tx = align + pix % 5 * horizontal_spacing
lix = lix + 1
local light = spawn("fx", self.level, self.x, self.y, 3, self.id.."_"..lix)
led[lix] = light.id
light:setLight(1, 0, 0, 1500, 0.24, 360000, false)
light:translate(tx * cos + tz * sin, height - math.floor(pix / 5) * vertical_spacing - 1, tz * cos + tx * sin * (1 - wall%2 * 2))
end
end
---
--- add one to the current display w/ wrap
---
function add()
display((displayed + 1)%100)
end
---
--- subtract one from the current display w/ wrap
---
function sub()
display((displayed + 99)%100)
end
---
--- turn lights off
---
function off()
if lix ~= nil and led ~= {nil} then
for dix = 1, lix do
local light = findEntity(led[dix])
if light ~= nil then light:destroy() end
end
end
lix = 0
led = {nil}
end
---
--- turn lights on
---
function on()
display(displayed)
end
---
--- turn lights on if specified in parameters
---
if initallyOn then on() end
Thank you, -Lark
Updated to version 1.4.1 on 10-10-2012
Updated to version 1.4.3 on 12-09-2014